Imagine we had a Lamp object in our scene, and a Switch we can flick so that it turns on. Both objects are instances of a Blueprint, so the switch needs to know which lamp we’re talking about. Although it seems obvious to us as humans (or to our 3D player), this situation isn’t as straightforward for a computer, unless we’re very specific about each object.
In this article I’ll show you how to reference one Blueprint from another in Unreal Engine. It’s not difficult, it’s all about knowing what to do where. In a nutshell we need
- a public property on the object we’re referencing (the Lamp in our example)
- a variable of type “other object” (the Lamp in our case, on the Switch object)
- a mechanism that sets the the variable on our target object from the source object
I won’t go into the details of the lamp or switch (like mesh, material or animation), only the principles or referencing instances in code. Let’s see how to do this step by step.
The Lamp Blueprint
I’m creating a boolean variable called isLightOn. This will be set to true if it’s lit, and false when it’s switched off (which it is by default). I’ll make sure it’s set to public so it can be read and set by other objects, like the light switch. Click the little eyeball icon to make it public.
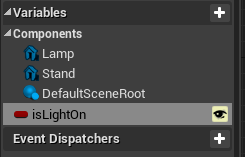
Somewhere in the Blueprint will be a mechanism that tests the value of this variable and reacts accordingly. An example is the Event Tick, which would check the state of the variable at every single rendered frame and decides what should happen. Something like this:
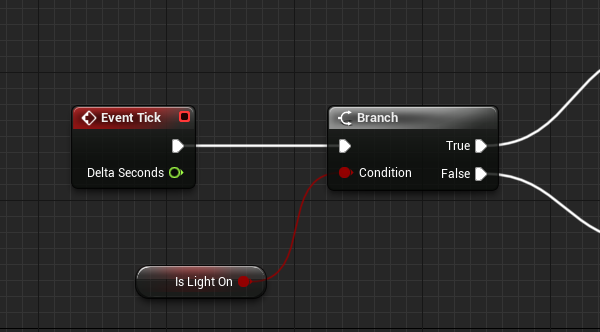
Compile and save before proceeding, otherwise the variable may not be accessible in the next step.
The Light Switch Blueprint
Meanwhile, our light switch object will have a mechanism to set this variable. To make that happen, we need to grab a reference to the object above. Let’s create a new variable in our Light Switch and call it Lamp. The default type is probably another boolean, or whatever type you’ve created before. Make sure it’s also set to public.
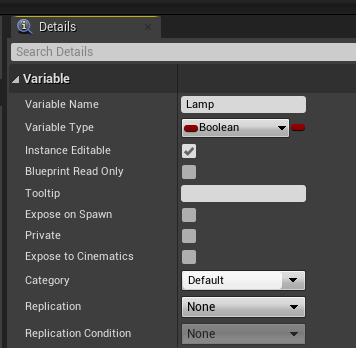
A boolean is not what we want, so let’s change it. Take a look at the Variable Type drop-down and search for the name you’ve given your previous Blueprint (i.e. the one you’d like to reference). In my case it’s a Lamp. As you pick it from the list, the variable type is changed to the object you’re referencing. This is seriously clever! It means we’ll have access to all that object’s public properties easily, such as our isLightOn variable.
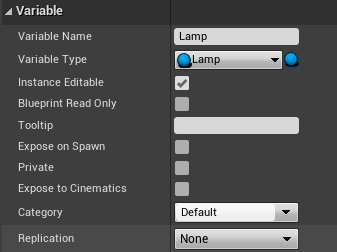
Since we’re reacting to a boolean, we can now branch off accordingly. We can turn the light on or off visually in our scene, and we must toggle the Lamp’s boolean variable so that it reflects our game world. Something like this:
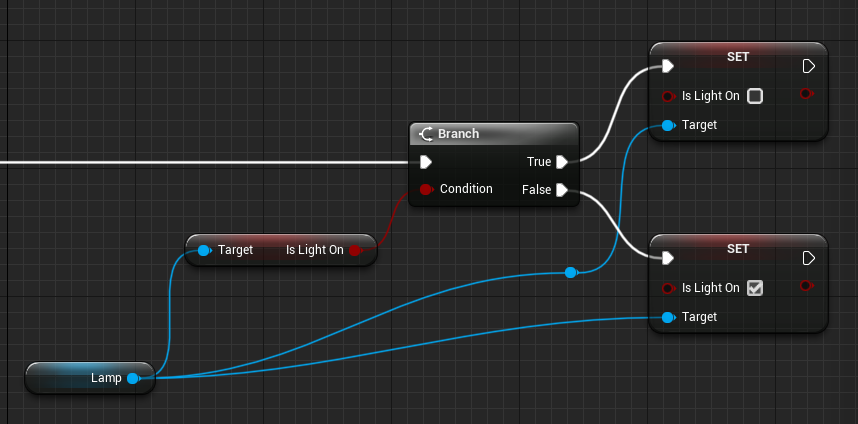
It looks a bit more complicated than it actually is: we’re grabbing a reference to our Lamp, on which we check the state of our boolean variable (search for “get is light on”). We’ll hook this up to a branch node, which then sets the value to the opposite of what it is (search for “set is light on”). Compile before proceeding to the next step.
Referencing the correct Lamp Instance
It is conceivable that we might drag several of our Lamp Blueprints into the scene, thereby creating various instances. Our switch needs to know which exact lamp we’re referring to. Even if there’s only a single Lamp instance, we need to tell it. This step is often overlooked, leading to compiler errors.
Thankfully we’ve set our Lamp variable on the Light Switch to public, so we can do this in the Details Panel. Find a section called Default and see the Lamp value exposed. Pick an item from the list, or use the eyedropper tool to pick your chosen lamp in the viewport.
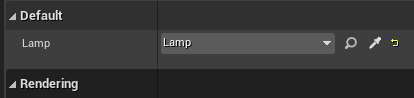
Congrats – you’ve successfully referenced one Blueprint from another! You can read more about this principle (called Cast To Referencing) in the Unreal Engine Documentation:
Hello, Nice Article.
what I want to do is that –
1. There is a Switch BP
2. There is a Lamp BP.
now whenever I drag a new instance of the Lamp to the level, it is already connected to the Switch BP. Which means all the Lamp instances react to the Switch at the same time. Which also means I am not assigning individual Lamps in the level but already assigned/referenced in the Switch BP itself. can you help?
I would tag the lamps, then on the switch blueprint, expose an option that lets you add that tag. Now you can marry up a particular switch and a particular lamp, or even multiple lamps if they have the same tag. You can use “Actor has Tag” to check and use a public Name variable for that. Hope this helps!