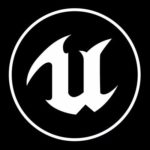
Interfaces are a C thing. They’re a set of functions that one class can implement so that an action is performed on another class of a potentially different type. Rather than calling a function explicitly on another class, we’re sending a message. If the receiving class knows what to do with it, it’ll react. If not, it’ll silently fail (rather than crash the whole programme). By this principle it is possible to have classes of different types react to the same message, each in their own way.
Interfaces are actually harder to explain than to use. Let me show you how to increase a game score using an interface call. I’ll let the Game Mode count the score, and every time a target is destroyed, the Target Class will send a message so the Game Mode can action it. In principle we need to
- create the interface
- define at least one function name in the interface (no code)
- tell our source class to use the interface (in this example: my Game Mode)
- implement (write code) in that class
- call the function from the another class (in this example: my Target)
Creating the Interface
The interface has a visual albeit minimal representation. It’s just a function definition without code. We can create one by right-clicking in our Content Browser (or by choosing the big green Add New button) and hovering over Blueprints, then picking Blueprint Interface. Give the interface a descriptive name, I’ll call mine Score Interface. It’ll have a snazzy icon to tell it apart from other file types.
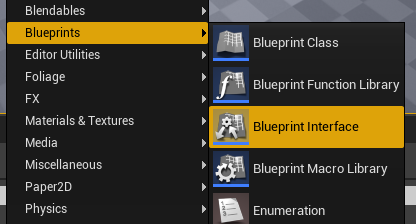
Double click to open the interface file. By default it will have a single function (called New Function 0), so let’s rename this to something more meaningful. We can do that on the top right. I’m calling my function Increase Score.
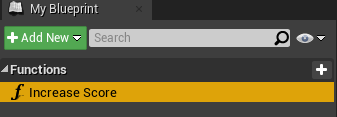
Define any input our output parameters at the bottom right if you need to. I’ll add an input integer called Score To Add. The whole screen looks a little forlorn, and we won’t be writing any code here. An interface definition is literally just that: defining the function name and any input/output parameters. Compile and close this screen, we’re done with it for now.
Writing the Function Code
I’d like my Game Mode to implement the interface. That’s done by heading over to Class Settings and adding the interface on the right hand side.
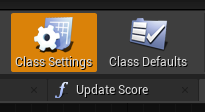
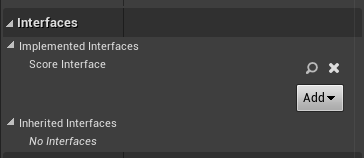
When done, the function we’ve defined in the interface file will be displayed on the left. We’ll have to write the code implementation next, so we’ll right-click on the function we’d like to code and choose Implement Function.
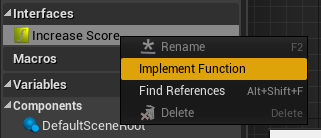
This will create an event that lets us nodes as usual. In my case, I’m adding an integer to an existing variable, thus increasing a score. If anyone in my game needs for this to happen, they’ll send a message and this class will action it.
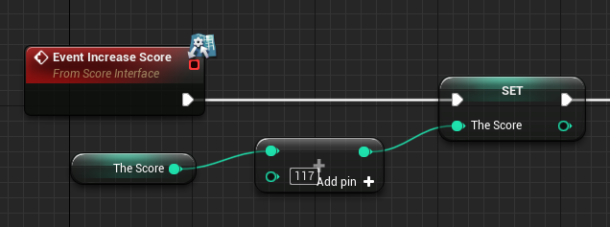
Notice the little arrow icons above the red event node. These indicate that this is an interface implementation.
Calling the Interface Function
In my example I’ll have a collectible object. When the player runs over it, the object will destroy itself. Before doing so though, it’ll tell the Game Mode to increase the score. So bit this is happening on any class that would like to have the score increased. Technically we’re sending a message “please update the score”, and if the referenced class knows how to do this, it’ll react.
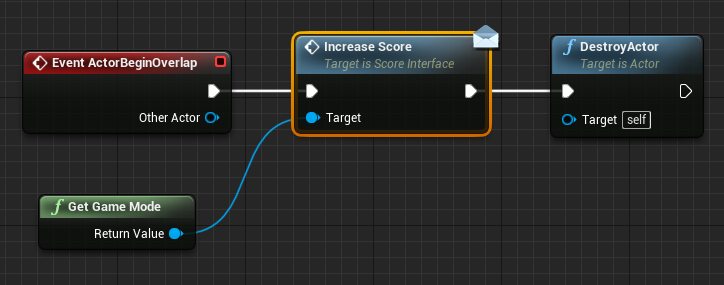
When someone collides with this object, I’ll get a reference to the Game Mode (the class implementing the interface). I’ll call the Increase Score function on it, then destroy my object. The little envelope icon indicates that this is not an explicit function call, but rather a message that’s sent, meaning if this class doesn’t implement the interface, the call will fail silently.
Note that I still need to reference the object I’m calling my function on (unlike in iOS, where a notification is sent out without a reference, and whoever is listening can react using observers).