The Tick Event is a somewhat special event that is called every time a frame is drawn in Unreal Engine. Many actions are derived from it in a running project. Sometimes we may need to drive things while we’re editing a project though, without being able to press play. Sadly we can’t use the Tick Event in that case, because it just isn’t called in the Editor.
Thankfully we can create our own version of an Editor Tick Event by creating a dummy class and calling a function repeatedly, giving us the same effect. It’s not exactly drag-and-drop, but once you’ve done it like 20 times it’ll soon grow on you. Thanks to SiLi for putting this video together! I thought I’d write the instructions up because the original source has gone down, and I’d like to remember how this works.
Here’s what we need to make this work:
- an Interface
- an Editor Ticker class
- any Blueprint in our project to implement the interface and call the ticker
We’ll do everything in Blueprint here, but there is a way to do this in C++, if you’re interested take a look at this code by artechorg.
The Interface
Create a new Blueprint Interface and call it BPI_EditorTick. It’ll only need a single function, let’s call it EditorTick. The only noteworthy thing here is that Call in Editor needs to be enabled. Compile, save and close the interface file, we won’t need it again.
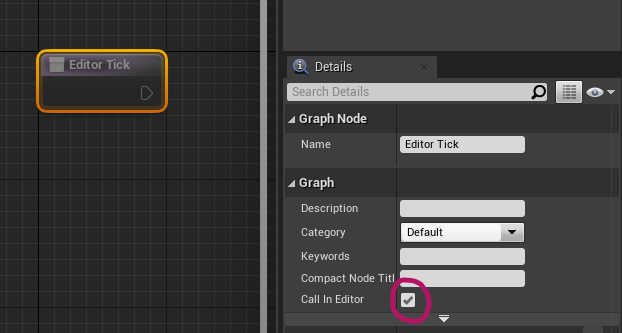
The Ticker Class
Create a new Blueprint Class and make it a child of the Editor Utility Actor class. Let’s call it BP_EditorTicker and enable the following parameters under Class Defaults. It’s easy to find them when you search for them:
- Allow Tick Before Begin Play
- Is Editor Only Actor
- Tick even when Paused
Let’s create a float variable and name it TickRatePerSecond. This is an optional adjustment that will allow us to throttle how many ticks are called. The default would be 60 (or rather “tick on every frame drawn”), but that’s often overkill. This variable will let us go easy on our system. I’ll set my default value to 30.
Next let’s head over to our Construction Script and string this network of nodes together. Click to enlarge:
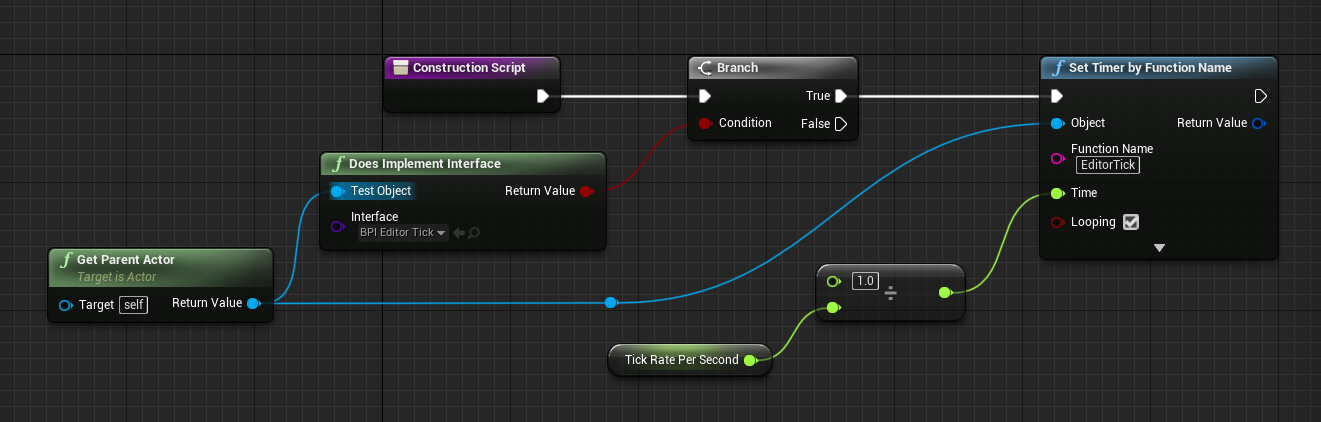
Here we grab our parent actor (i.e. the Blueprint we want to use our Editor Ticker from) and check if it implements our BPI_Editor Tick interface. If it does, we create a timer by function event and call the EditorTick function at a rate specified by our Tick Rate variable. We have to divide 1 by our variable as it expects the time in seconds. Make sure looping is enabled, and don’t forget to specify the interface and function name correctly.
Compile and save, we’re done with this class.
Hooking in to our Editor Ticker
Open the event graph for the class you’d like to use with this Editor Ticker event. Switch over to Class Settings and implement the BPI_EditorTick interface.
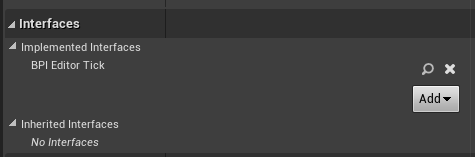
Head over to the Construction Script and grab an Add Child Actor Component class node. With it selected, pick the BP_EditorTicker class from the drop down as child actor class. This will spawn our ticker class as soon as this class is added to our scene.
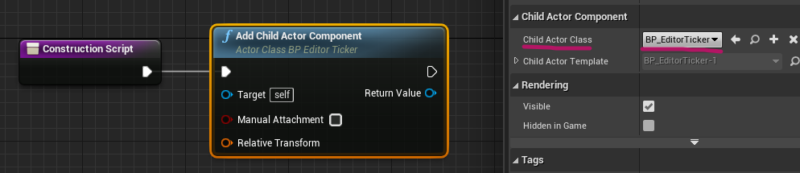
Now head over to the main event graph, grab the Event Editor Tick and hook it up to whatever needs to be done.
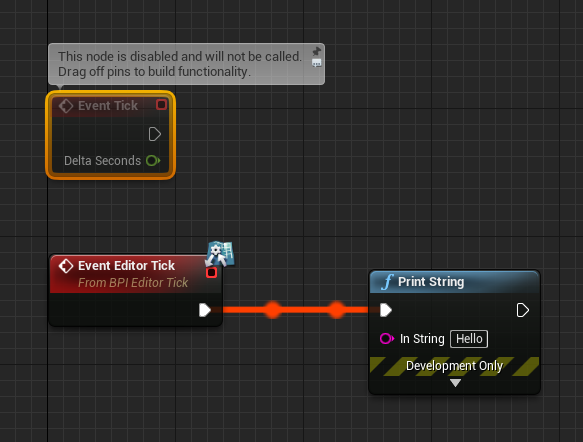
If all went well, your action should be called repeatedly in the viewport without running the game. If it doesn’t work, you made a mistake somewhere (I had to build this 5 times to get it right, so have faith).
GitHub Repository
If you want to get going with a working version I’ve made in Unreal Engine 4.26, download the fully working demo project here: